INSERTING LIST OF RECORDS IN A SiNGLE CLICK
Managing a huge number of database records related to a particular entity is very difficult in today's modern applications. For example,one customer can purchase multiple types of items, so in this case case the customer is a single entity and the items he is purchasing are different entities having multiple records with dynamic entries where we don't know the count. So in this scenario it's very difficult to manage the database table structure, so this article is related to Solve this issue -
So, let's learn step by step so beginners can also learn how to Insert Bulk Record in A Single Click
Step 1: Create an MVC Application.
Now let us start with a step by step approach from the creation of a simple MVC application as in the following:
- create a New ASPMVC4 Application Named "MVCApplication7"
- Go t File->New->Project->ASP.NET MVC4 Web Application .Then Provide the Project Name as you wish.After clicking, the following window will appear :
- As shown in the preceding screenshot, click on the Basic template, then click OK. This will create an empty MVC web application.
Step 2: Add The Reference of Dapper ORM into Project.
Now the next step is to add the reference of Dapper ORM into our created MVC Project. Here are the steps:
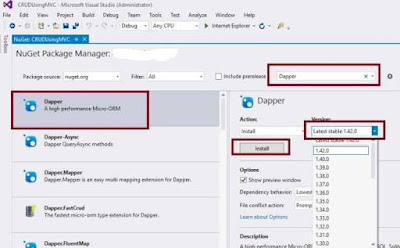
Now the next step is to add the reference of Dapper ORM into our created MVC Project. Here are the steps:
Right click on Solution, find Manage NuGet Package manager and click on it.
- After as shown in the image and type in search box "dapper".
- Select Dapper as shown in the image.
- Choose the version of the dapper library and click on install button.
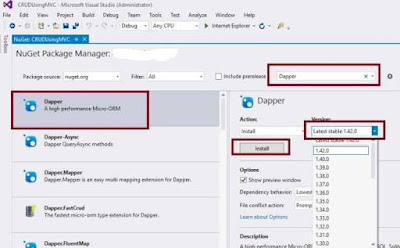
After installing the Dapper library, it will be added into the References of our solution explorer of MVC application such as:
Step 3: Create Model Class.
Now let's create the model class named ProductModel.cs by right-clicking on model folder and
Now let's create the model class named ProductModel.cs by right-clicking on model folder and
also Create one More Class To Map Customers With Ordered Product - EmployeeModel.cs
Go to Solution Explorer -> Right Click on Models Folder ->Add -> Class ->Write the Name of class “ProductModel.cs”.
Note:
It is not mandatory that Model class should be in Model folder, it is just for better readability you can create this class anywhere in the solution explorer. This can be done by creating different folder names or without the folder name or in a separate class library.
It is not mandatory that Model class should be in Model folder, it is just for better readability you can create this class anywhere in the solution explorer. This can be done by creating different folder names or without the folder name or in a separate class library.
- using System;
- using System.Collections.Generic;
- using System.ComponentModel.DataAnnotations;
- namespace MVCApplication7.Models
- {
- public class EmployeeModel
- {
- public int ID { get; set; }
- public string Name { get; set; }
- public int Age { get; set; }
- public List<ProductModel>Products { get; set; }
- }
- public class ProductModel
- {
- public int ID{get;set;}
- public string ProductName{get;set;}
- public int ProductCost{ get; set; }
- }
- }
Step 4 : Create Controller.
Now let us add the MVC 5 controller "HomeController"
Now let us add the MVC 5 controller "HomeController"
Go to Solution Explorer -> Right Click on Controllers Folder ->Add -> Controller ->Write the Name of Controller “HomeController”.
Note: The controller name must have the suffix as 'Controller' after specifying the name of controller.
Create Table and Stored procedure.
Step 5 : Now before creating the views let us create the table name customers , CustomerProduct in database to store the order details:
I hope you have created the same table structure as shown above. Now create the stored procedures to insert the order details as in the following code snippet:
1-Here I am Creating AddCustomer Procedure to Add Customer Record in Customers Table
- Create procedure AddCustmer
- (
- @Name varchar(50),
- @Age int,
- @ID int =null output
- as
- Begin
- INSERT INTO [dbo].[Customers]
- (
- Name,Age
- )
- VALUES
- (
- @Name,
- @Age
- )
- set @ID=SCOPE_IDENTITY()
- return @id
- End
2-Here I am Creating AddCustomer Procedure to Add Products Record in Customers Table
- Create procedure AddCustomerProduct
- (
- @Name varchar(50),
- @Cost int,
- @ID int =null
- as
- Begin
- INSERT INTO [dbo].[CustomerProduct]
- (
- ID,ProductName,ProductCost
- )
- VALUES
- (
- @ID,
- @Name,
- @Cost
- )
- End
Step 6: Create Repository class.
Go to Solution Explorer -> Right Click on Models Folder ->Add -> Class ->Write the Name of Class “DataLayer”.
- using System;
- using System.Collections.Generic;
- using System.Linq;
- using System.Web;
- using System.Data.SqlClient;
- using System.Configuration;
- using Dapper;
- using System.Data;
- using System.Web.Mvc;
- namespace MvcApplication7.Models
- {
- public class DataLayer
- {
- SqlConnection Con;
- public DataLayer()
- {
- Connectin();
- }
- private void Connectin()
- {
- Con = new SqlConnection("Data Source=Dhananjay\SqlExpress;User Id=sa;Password=1;Initial Catalog=Dhananjay");
- if (Con.State == System.Data.ConnectionState.Open)
- {
- Con.Close();
- }
- Con.Open();
- }
- public bool AddCustomer(EmployeeModel E)
- {
- try
- {
- DynamicParameters Parm = new DynamicParameters();
- Parm.Add("@Name", E.Name);
- Parm.Add("@Age", E.Age);
- Parm.Add("@id", dbType: DbType.Int32, direction: ParameterDirection.Output, size: 5215585);
- Con.Execute("AddCustmer", Parm, commandType: System.Data.CommandType.StoredProcedure);
- var ProductID = Parm.Get<Int32>("@id");
- foreach(var p in E.Products)
- {
- DynamicParameters Parm2 = new DynamicParameters();
- Parm2.Add("@ID", ProductID);
- Parm2.Add("@Name", p.ProductName);
- Parm2.Add("@Cost", p.ProductCost);
- Con.Execute("ADdCustomerProduct", Parm2, commandType: System.Data.CommandType.StoredProcedure);
- }
- return true;
- }
- catch (Exception c) { return false; }
- }
- }
- }
Step 7: Create Method into the HomeController.cs file.
Now open the HomeController.cs and create the following action methods:
Now open the HomeController.cs and create the following action methods:
- using System.Collections.Generic;
- using System.Web.Mvc;
- using System;
- using System.Linq;
- using System.Web;
- using MvcApplicatin7.Models;
- namespace MvcApplication7.Controllers
- {
- public class CustomerController : Controller
- {
- // GET: Customer
- public ActionResult AddProduct()
- {
- return View();
- }
- [HttpPost]
- public ActionResult AddProduct(EmployeeModel E)
- {
- return View(new DataLayer().AddCustomer(E));
- }
- }
- }
Step 8 : Create view named AddProduct
- @{
- ViewBag.Title = "AddProduct";
- }
- <h2>AddProduct</h2>
- <script src="~/Scripts/jquery-1.7.1.min.js"></script>
- <script>
- $(document).ready(function () {
- $('input[type="text"].num').bind('keydown', function (e) {
- // Allow: backspace, delete, tab, escape, enter and .
- if ($.inArray(e.keyCode, [46, 8, 9, 27, 13, 110, 190]) !== -1 ||
- // Allow: Ctrl+A
- (e.keyCode == 65 && e.ctrlKey === true) ||
- // Allow: Ctrl+C
- (e.keyCode == 67 && e.ctrlKey === true) ||
- // Allow: Ctrl+X
- (e.keyCode == 88 && e.ctrlKey === true) ||
- // Allow: home, end, left, right
- (e.keyCode >= 35 && e.keyCode <= 39)) {
- // let it happen, don't do anything
- return;
- }
- if ((e.shiftKey || (e.keyCode < 48 || e.keyCode > 57)) && (e.keyCode < 96 || e.keyCode > 105)) {
- e.preventDefault();
- }
- });
- });
- function AddMore() {
- $("#Products tr:last").after('<tr det="Product"><td><input type="text"></td><td><input type="text"></td></tr>');
- }
- function Add() {
- debugger;
- var OrderedProducts = [];
- var isOk = true;
- $('#Products tr[det="Product"]').each(function () {
- var Product = $($(this).find('input[type="text"]')[0]).val();
- var Cost = parseFloat($($(this).find('input[type="text"]')[1]).val());
- OrderedProducts.push({
- ProductName: Product,
- ProductCost: parseInt(Cost),
- });
- })
- if (!isOk) {
- return;
- }
- $('input[type^="text"]').each(function () {
- if ($(this).val() == '') {
- alert('Please Enter ' + $(this).parent().prev().text());
- $(this).focus();
- isOk = false;
- return false;
- }
- });
- if (!isOk) {
- return;
- }
- var Order =
- {
- PurchaseOrderNo: $('#txtPONo').val(),
- Name: $('#UName').val(),
- Age: $('#UAge').val(),
- Products: OrderedProducts
- };
- debugger;
- $.ajax({
- type: "POST",
- dataType: 'text',
- contentType: 'application/json; charset=utf-8',
- url: "/Home/AddProduct",
- data: JSON.stringify(Order),
- success: function (res) {
- if (res == "True") {
- alert('Information Saved Successfully!');
- window.location.reload();
- }
- else {
- alert('Some Error occurred!');
- }
- },
- error: function (xhr, textStatus, errorThrown) {
- alert('Some Error occurred!');
- }
- });
- }
- </script>
- <table>
- <tr>
- <td>Name</td>
- <td><input type="text" id="UName" /></td>
- </tr>
- <tr>
- <td>Age</td>
- <td><input type="text" id="UAge" class="num" /></td>
- </tr>
- </table>
- <table id="Products">
- <tr>
- <th>Product Name</th>
- <th>Product Cost</th>
- <th></th>
- </tr>
- <tr det="Product">
- <td><input type="text" /></td>
- <td><input type="text" class="num" /></td>
- </tr>
- </table>
- <input type="button" onclick="AddMore()" value="Add" />
- <input type="button" value="Click" onclick="Add()" />
Step 09: Now run the application