SENDING EMAILS IN ASP.NET MVC FROM CONTROLLER USING WEBMAIL HELPER Part 2
- Active internet connection.
- Email id of any provider such as Gmail, Yahoo or your organization to send emails.
- "Start", then "All Programs" and select "Microsoft Visual Studio 2015".
- "File", then "New" and click "Project", then select "ASP.NET Web Application Template", then provide the Project a name as you wish and click OK. After clicking, the following window will appear:
In my previous article on Sending Emails In ASP.NET MVC From Razor View, we have
learned how to send emails from View using WebMail Class , Now in this article
we will learn how to send emails in ASP.NET MVC from controller with the help
WebMail helper class. So let's learn step by step so beginners can also learn
how to send emails in ASP.NET MVC from
controller.
Prerequisites
Now
let's create a simple MVC application to demonstrate this.
Step 1: Create an MVC Application.
Now
let us start with a step by step approach from the creation of a simple MVC
application as in the following:
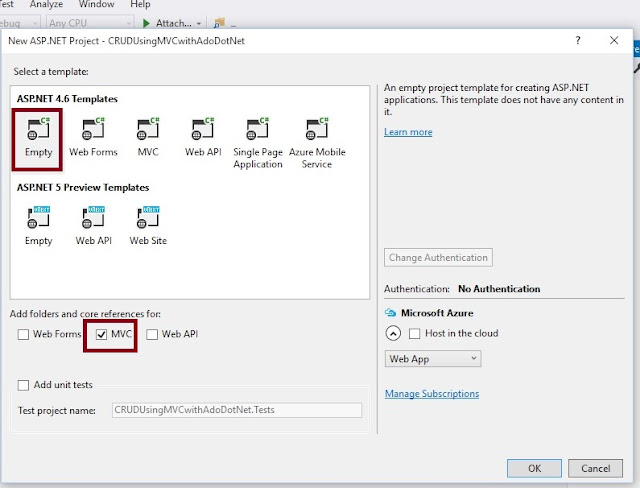
- using System.ComponentModel.DataAnnotations;
- namespace SendingEmailsFromController.Models
- {
- public class EmployeeModel
- {
- [DataType(DataType.EmailAddress),Display(Name ="To")]
- [Required]
- public string ToEmail { get; set; }
- [Display(Name ="Body")]
- [DataType(DataType.MultilineText)]
- public string EMailBody { get; set; }
- [Display(Name ="Subject")]
- public string EmailSubject { get; set; }
- [DataType(DataType.EmailAddress)]
- [Display(Name ="CC")]
- public string EmailCC { get; set; }
- [DataType(DataType.EmailAddress)]
- [Display(Name ="BCC")]
- public string EmailBCC { get; set; }
- }
- }
- using SendingEmailsFromController.Models;
- using System;
- using System.Web.Helpers;
- using System.Web.Mvc;
- namespace SendingEmailsFromController.Controllers
- {
- public class HomeController : Controller
- {
- // GET: Home
- public ActionResult SendEmail()
- {
- return View();
- }
- [HttpPost]
- public ActionResult SendEmail(EmployeeModel obj)
- {
- try
- {
- //Configuring webMail class to send emails
- //gmail smtp server
- WebMail.SmtpServer = "smtp.gmail.com";
- //gmail port to send emails
- WebMail.SmtpPort = 587;
- WebMail.SmtpUseDefaultCredentials = true;
- //sending emails with secure protocol
- WebMail.EnableSsl = true;
- //EmailId used to send emails from application
- WebMail.UserName = "YourGamilId@gmail.com";
- WebMail.Password = "YourGmailPassword";
- //Sender email address.
- WebMail.From = "SenderGamilId@gmail.com";
- //Send email
- WebMail.Send(to: obj.ToEmail, subject: obj.EmailSubject, body: obj.EMailBody, cc: obj.EmailCC, bcc: obj.EmailBCC, isBodyHtml: true);
- ViewBag.Status = "Email Sent Successfully.";
- }
- catch (Exception)
- {
- ViewBag.Status = "Problem while sending email, Please check details.";
- }
- return View();
- }
- }
- }
- @model SendingEmailsFromController.Models.EmployeeModel
- @{
- ViewBag.Title = "www.compilemode.com";
- }
- @using (Html.BeginForm())
- {
- @Html.AntiForgeryToken()
- <div class="form-horizontal">
- <hr />
- @Html.ValidationSummary(true, "", new { @class = "text-danger" })
- <div class="form-group">
- @Html.LabelFor(model => model.ToEmail, htmlAttributes: new { @class = "control-label col-md-2" })
- <div class="col-md-10">
- @Html.EditorFor(model => model.ToEmail, new { htmlAttributes = new { @class = "form-control" } })
- @Html.ValidationMessageFor(model => model.ToEmail, "", new { @class = "text-danger" })
- </div>
- </div>
- <div class="form-group">
- @Html.LabelFor(model => model.EMailBody, htmlAttributes: new { @class = "control-label col-md-2" })
- <div class="col-md-10">
- @Html.EditorFor(model => model.EMailBody, new { htmlAttributes = new { @class = "form-control" } })
- @Html.ValidationMessageFor(model => model.EMailBody, "", new { @class = "text-danger" })
- </div>
- </div>
- <div class="form-group">
- @Html.LabelFor(model => model.EmailSubject, htmlAttributes: new { @class = "control-label col-md-2" })
- <div class="col-md-10">
- @Html.EditorFor(model => model.EmailSubject, new { htmlAttributes = new { @class = "form-control" } })
- @Html.ValidationMessageFor(model => model.EmailSubject, "", new { @class = "text-danger" })
- </div>
- </div>
- <div class="form-group">
- @Html.LabelFor(model => model.EmailCC, htmlAttributes: new { @class = "control-label col-md-2" })
- <div class="col-md-10">
- @Html.EditorFor(model => model.EmailCC, new { htmlAttributes = new { @class = "form-control" } })
- @Html.ValidationMessageFor(model => model.EmailCC, "", new { @class = "text-danger" })
- </div>
- </div>
- <div class="form-group">
- @Html.LabelFor(model => model.EmailBCC, htmlAttributes: new { @class = "control-label col-md-2" })
- <div class="col-md-10">
- @Html.EditorFor(model => model.EmailBCC, new { htmlAttributes = new { @class = "form-control" } })
- @Html.ValidationMessageFor(model => model.EmailBCC, "", new { @class = "text-danger" })
- </div>
- </div>
- <div class="form-group">
- <div class="col-md-offset-2 col-md-10">
- <input type="submit" value="Send" class="btn btn-primary" />
- </div>
- </div>
- <div class="form-group">
- <div class="col-md-offset-2 col-md-10 text-success">
- @ViewBag.Status
- </div>
- </div>
- </div>
- }
3. As
shown in the preceding screenshot, click on Empty template
and check MVC option, then
click OK. This will create an
empty MVC web application whose Solution Explorer will look like the
following:
Step 2: Create Model Class.
Now
let us create the model class named EmployeeModel.cs by right clicking on
model folder as in the following screenshot:
Note:
It is
not mandatory that Model class should be in Model folder, it is just for
better readability you can create this class anywhere in the solution
explorer. This can be done by creating different folder names or without
folder name or in a separate class library.
EmployeeModel.cs
class code snippet:
Step 3 : Add Controller Class.
Now
let us add the MVC 5 controller as in the following screenshot:
After
clicking on Add button it
will show the window. specify the Controller
name as Home with suffix Controller.
Note:
The
controller name must be having suffix as 'Controller' after specifying the
name of controller. Now the default code in HomeController.cs will look like
as follows,
HomeController.cs
Step 4 :
Create
strongly typed view named SendEmail using EmployeeModel class.
Right
click on View folder of created application and choose add view , select
employee model class and scaffolding template as create . As shown in the
following image,
Now
open the SendEmail.cshtml view , Then following default code you will see
which is generated by MVC scaffolding template as,
SendEmail.cshtml
Now
after adding the model , view and controller our application solution explorer
will look as follows,
Now
we have done all coding to send emails using WebMail class.
Step 5 : Now run the application.
No comments:
Post a Comment